1
/
of
1
MACAUBET ♻️ Daftar Situs Slot Hoki Gacor Terbaik MACAUBET Winrate Up To 99%
MACAUBET ♻️ Daftar Situs Slot Hoki Gacor Terbaik MACAUBET Winrate Up To 99%
Regular price
Rp 20.000,00 IDR
Regular price
Sale price
Rp 20.000,00 IDR
Unit price
/
per
Couldn't load pickup availability
MACAUBET MACAUBET adalah situs slot gacor yang hoki nya besar, MACAUBET mempunyai RTP live slot yang sangat tinggi dan gampang menang.
Share
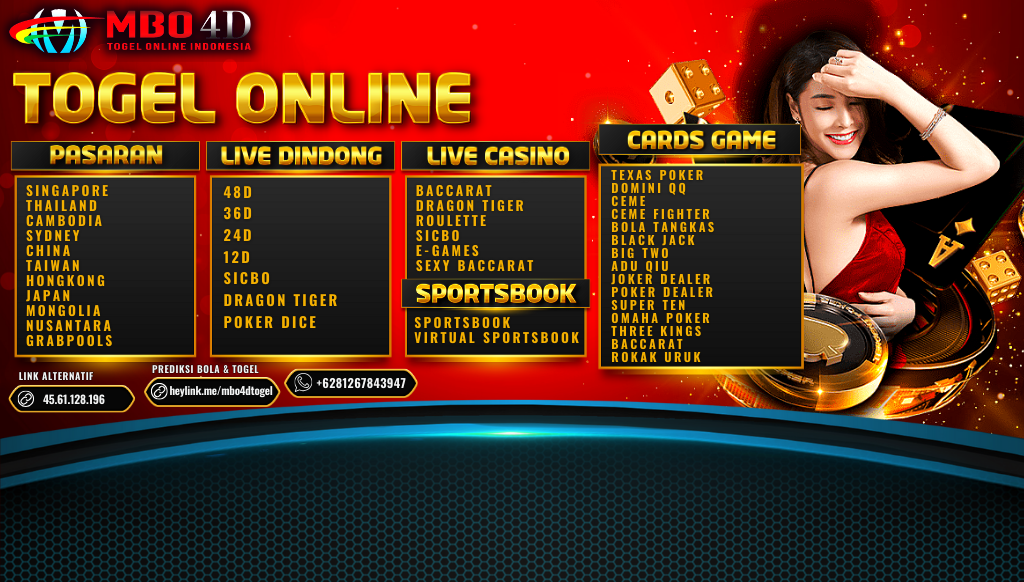